Build agentic automations. Fast.
Connect LLMs to 13,000+ APIs with human-verifiable calls, streaming execution, and SDKs for LangChain, Vercel, and more.
1from langchain_openai import ChatOpenAI
2from langchain.agents import AgentType
3from pica_langchain import PicaClient, create_pica_agent
4
5# Initialize Pica client
6pica_client = PicaClient(secret="YOUR_PICA_SECRET", connectors=["*"])
7
8# Set up LLM
9llm = ChatOpenAI(temperature=0, model="gpt-4o")
10
11# Create agent with Pica tools
12agent = create_pica_agent(
13 client=pica_client,
14 llm=llm,
15 agent_type=AgentType.OPENAI_FUNCTIONS,
16 verbose=True
17)
18
19# Execute a multi-step workflow
20result = agent.invoke({
21 "input": "Star the picahq/pica repo in github "
22 "Then, list the number of stars for the picahq/pica repo in github."
23})
24
25print(f"Result: {result}")
26
LangChain integration for AI agents.
Product Features
Everything you need to build powerful agentic applications
LLM-First
Built from the ground up for large language models and AI agents
13,000+ APIs
Connect to thousands of services with natural language
Fast & Reliable
Streaming execution with human-verifiable calls
Open + Extensible
SDKs for LangChain, Vercel, and more
See Pica in Action
Watch how Pica transforms natural language into powerful automations
Products
Three tools, one goal: `Get real work done fast.`

Call any API with a single install. OneTool gives your agent instant access to every integration, hassle-free.
- Full API coverage for every integration.
- Schemas ready to use out of the box.
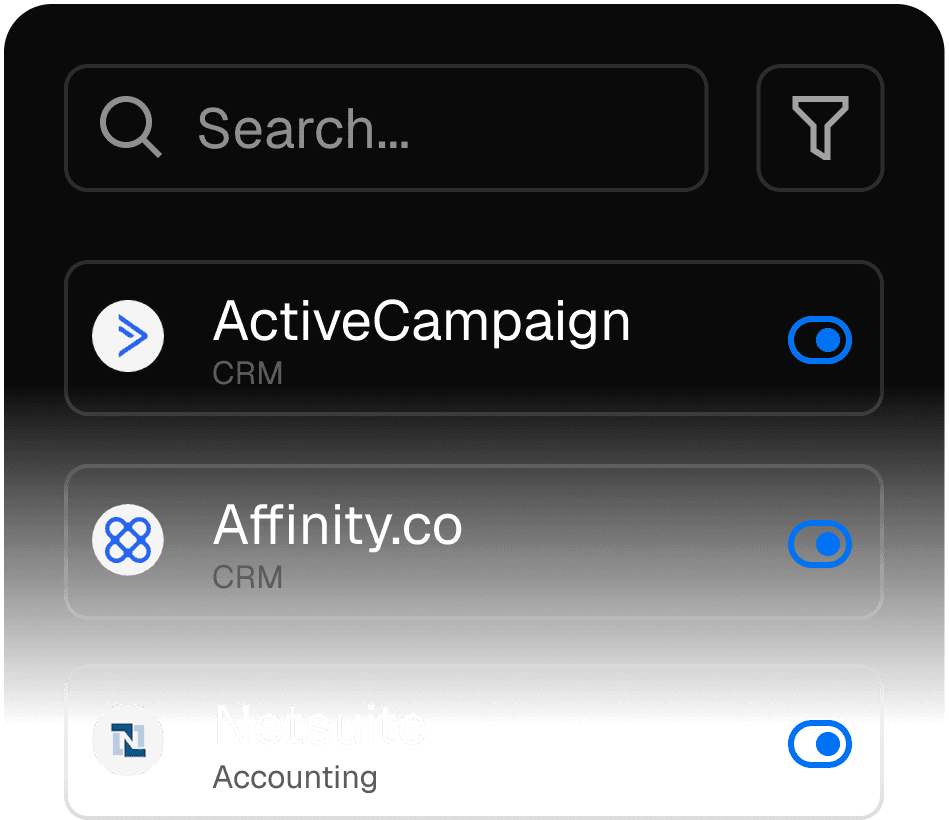
Authenticate users and manage tokens effortlessly. AuthKit handles OAuth and token security so you don't have to.
- OAuth handled end-to-end.
- Tokens stored and rotated for you.

Describe what you want to build and BuildKit creates the integration for you—no docs or configs needed.
- API docs and schemas synced daily.
- Changes flagged before they break.
MCP App Store-as-a-Service
Launch and manage internal AI tools with full control.
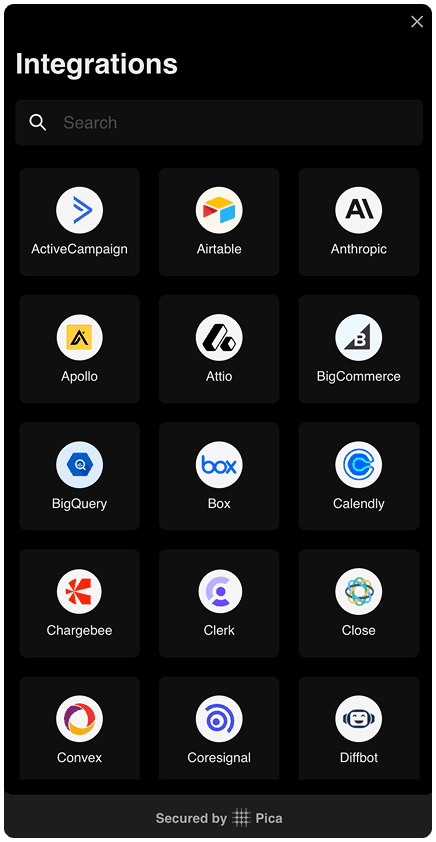
1import { useAuthKit } from "@picahq/authkit";
2import { Button } from "@/components/ui/button";
3
4export function AuthKitButton() {
5 const { open } = useAuthKit({
6 token: {
7 url: "https://api.your-company-name.com/authkit-token",
8 headers: {},
9 },
10 onSuccess: (connection) => {},
11 onError: (error) => {},
12 onClose: () => {},
13 });
14
15 return (
16 <Button onClick={open}>
17 Connect Tools
18 </Button>
19 );
20}